我們已經看到了組件和它的使用。例如,我們有一個需要在整個項目中重用的內容。我們可以將其轉換爲組件並使用它。
讓我們看一個簡單組件的例子,看看render函數在其中要做什麼。
Example
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "component_test"> <testcomponent></testcomponent> </div> <script type = "text/javascript"> Vue.component('testcomponent',{ template : '<h1>Hello World</h1>', data: function() { }, methods:{ } }); var vm = new Vue({ el: '#component_test' }); </script> </body> </html>
考慮上面一個列印Hello World的簡單組件的例子,如下面的螢幕截圖所示。
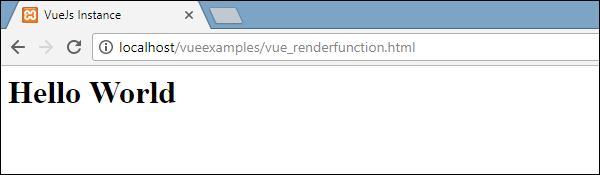
現在,如果我們想重用組件,只需再次列印即可。例如,
<div id = "component_test"> <testcomponent></testcomponent> <testcomponent></testcomponent> <testcomponent></testcomponent> <testcomponent></testcomponent> </div>
輸出如下。
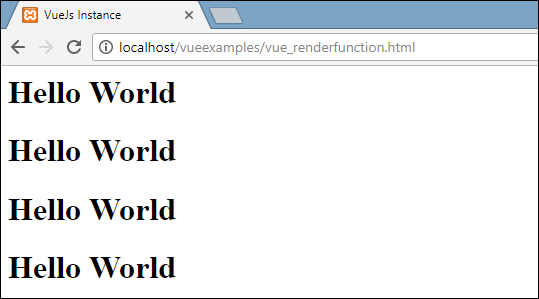
但是,現在我們需要對組件進行一些更改。我們不希望列印相同的文本。我們怎樣才能改變它?萬一,我們在組件中鍵入一些內容,會考慮到嗎?
讓我們考慮下面的例子,看看會發生什麼。
<div id = "component_test"> <testcomponent>Hello Jai</testcomponent> <testcomponent>Hello Roy</testcomponent> <testcomponent>Hello Ria</testcomponent> <testcomponent>Hello Ben</testcomponent> </div>
產出與我們之前看到的一樣。它並沒有按我們的要求更改文本。
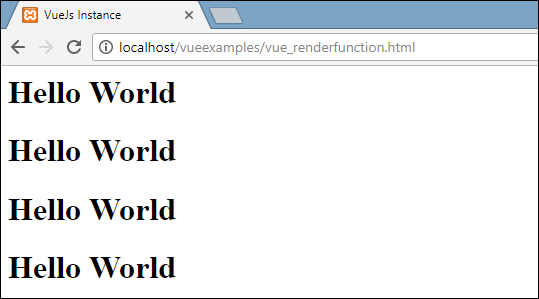
組件確實提供了稱爲插槽的功能。讓我們好好利用它看看是否能達到預期的效果。
Example
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "component_test"> <testcomponent>Hello Jai</testcomponent> <testcomponent>Hello Roy</testcomponent> <testcomponent>Hello Ria</testcomponent> <testcomponent>Hello Ben</testcomponent> </div> <script type = "text/javascript"> Vue.component('testcomponent',{ template : '<h1><slot></slot></h1>', data: function() { }, methods:{ } }); var vm = new Vue({ el: '#component_test' }); </script> </body> </html>
如上面的代碼所示,在模板中我們添加了slot,因此現在需要將值發送到組件內部,如下面的螢幕截圖所示。
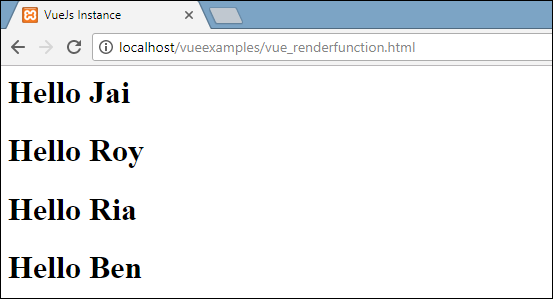
現在,讓我們考慮一下我們想改變顏色和尺寸。例如,目前我們正在使用h1標記,我們希望將同一組件的HTML標記更改爲p標記或div標記。我們怎麼能有這麼多的靈活性來進行這麼多的改變呢?
我們可以在render函數的幫助下這樣做。Render函數通過保持組件的通用性並幫助使用同一組件傳遞參數,有助於使組件動態化並按需要的方式使用它。
Example
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "component_test"> <testcomponent :elementtype = "'div,red,25,div1'">Hello Jai</testcomponent> <testcomponent :elementtype = "'h3,green,25,h3tag'">Hello Roy</testcomponent> <testcomponent :elementtype = "'p,blue,25,ptag'">Hello Ria</testcomponent> <testcomponent :elementtype = "'div,green,25,divtag'">Hello Ben</testcomponent> </div> <script type = "text/javascript"> Vue.component('testcomponent',{ render :function(createElement){ var a = this.elementtype.split(","); return createElement(a[0],{ attrs:{ id:a[3], style:"color:"+a[1]+";font-size:"+a[2]+";" } }, this.$slots.default ) }, props:{ elementtype:{ attributes:String, required:true } } }); var vm = new Vue({ el: '#component_test' }); </script> </body> </html>
在上面的代碼中,我們已經更改了組件,並使用下面的代碼添加了render函數with props屬性。
Vue.component('testcomponent',{ render :function(createElement){ var a = this.elementtype.split(","); return createElement(a[0],{ attrs:{ id:a[3], style:"color:"+a[1]+";font-size:"+a[2]+";" } }, this.$slots.default ) }, props:{ elementtype:{ attributes:String, required:true } } });
道具如下。
props:{ elementtype:{ attributes:String, required:true } }
我們定義了一個名爲elementtype的屬性,它接受類型爲string的attributes欄位。另一個必需欄位,其中提到該欄位是必需的。
在render函數中,我們使用了elementtype屬性,如下面的代碼所示。
render :function(createElement){ var a = this.elementtype.split(","); return createElement(a[0],{ attrs:{ id:a[3], style:"color:"+a[1]+";font-size:"+a[2]+";" } }, this.$slots.default ) }
Render函數將createElement作爲參數並返回相同的值。CreateElement創建DOM元素的方式與在JavaScript中相同。我們還使用attrs欄位中的值在逗號上拆分了elementtype。
CreateElement將第一個參數作爲要創建的elementtag。它使用以下代碼傳遞給組件。
<testcomponent :elementtype = "'div,red,25,div1'">Hello Jai</testcomponent>
組件需要使用上面顯示的props欄位。開頭是:還有道具的名字。在這裡,我們傳遞元素標籤、顏色、字體大小和元素的id。
在render函數中,在createElement中,我們是用逗號分隔的,因此第一個元素是elementtag,它被賦予createElemet,如下一段代碼所示。
return createElement( a[0],{ attrs:{ id:a[3], style:"color:"+a[1]+";font-size:"+a[2]+";" } }, this.$slots.default )
a[0]是html元素標記。下一個參數是元素標記的屬性。它們在下面代碼的attr欄位中定義。
attrs:{ id:a[3], style:"color:"+a[1]+";font-size:"+a[2]+";" }
我們爲元素標記定義了兩個屬性-id和style。對於id,我們傳遞一個[3],這是我們在逗號上拆分後得到的值。使用樣式,我們定義了顏色和字體大小。
最後一個是插槽,這是我們在下面的代碼中在組件中給出的消息。
<testcomponent :elementtype = "'div,red,25,div1'">Hello Jai</testcomponent>
我們使用下面的代碼定義了要在createElement中列印的文本。
this.$slots.default
它採用組件欄位中指定的默認值。
下面是我們在瀏覽器中得到的輸出。
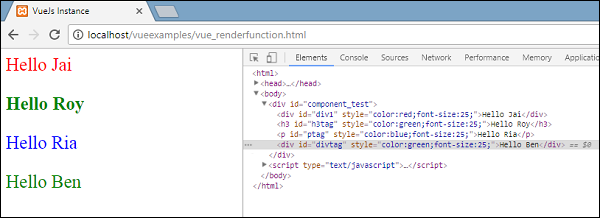
元素也顯示了結構。這些是我們定義的組件;