要從VueJS開始,我們需要創建Vue的實例,稱爲根Vue實例。
Syntax
var app = new Vue({ // options })
讓我們看一個例子來了解什麼需要成爲Vue構造函數的一部分。
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "vue_det"> <h1>Firstname : {{firstname}}</h1> <h1>Lastname : {{lastname}}</h1> <h1>{{mydetails()}}</h1> </div> <script type = "text/javascript" src = "js/vue_instance.js"></script> </body> </html>
vue_instance.js
var vm = new Vue({ el: '#vue_det', data: { firstname : "Ria", lastname : "Singh", address : "Mumbai" }, methods: { mydetails : function() { return "I am "+this.firstname +" "+ this.lastname; } } })
對於Vue,有一個名爲el的參數。它接受DOM元素的id。在上面的例子中,我們有id#vue_det。它是div元素的id,它出現在.html中。
<div id = "vue_det"></div>
現在,無論我們要做什麼,都將影響div元素,並且不會影響它之外的任何內容。
接下來,我們定義了數據對象。它有值firstname、lastname和address。
在div中分配相同的值。例如,
<div id = "vue_det"> <h1>Firstname : {{firstname}}</h1> <h1>Lastname : {{lastname}}</h1> </div>
Firstname:{{Firstname}值將在插值中被替換,即{{}替換爲在數據對象(即Ria)中分配的值。姓氏也一樣。
接下來,我們有定義函數mydetails和返回值的方法。在div中指定爲
<h1>{{mydetails()}}</h1>
因此,在{{}}內部調用函數mydetails。Vue實例中返回的值將在{{}}中列印。檢查輸出以供參考。
Output
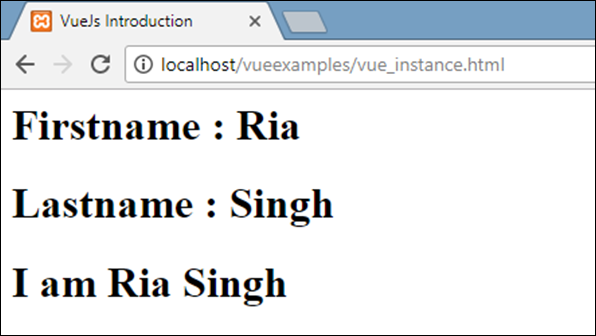
現在,我們需要將選項傳遞給Vue構造函數,它主要是數據、模板、要裝載的元素、方法、回調等。
讓我們看看傳遞給Vue的選項。
#數據−此類數據可以是對象或函數。Vue將其屬性轉換爲getter/setter以使其具有反應性。
讓我們看看如何在選項中傳遞數據。
Example
<html> <head> <title>VueJs Introduction</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <script type = "text/javascript"> var _obj = { fname: "Raj", lname: "Singh"} // direct instance creation var vm = new Vue({ data: _obj }); console.log(vm.fname); console.log(vm.$data); console.log(vm.$data.fname); </script> </body> </html>
Output
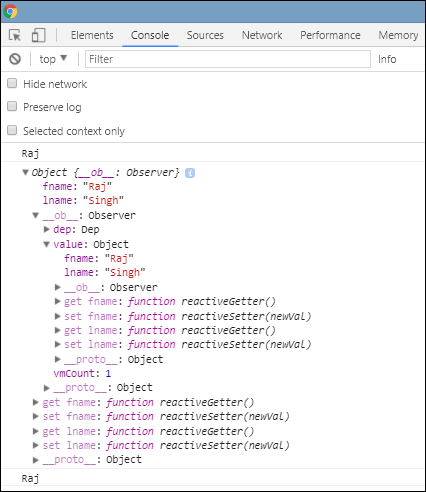
console.log(vm.fname);/列印Raj
console.log(vm.$data);如上圖所示列印完整對象
console.log(vm.$data.fname);//列印Raj
如果存在組件,則必須從函數引用數據對象,如以下代碼所示。
<html> <head> <title>VueJs Introduction</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <script type = "text/javascript"> var _obj = { fname: "Raj", lname: "Singh"}; // direct instance creation var vm = new Vue({ data: _obj }); console.log(vm.fname); console.log(vm.$data); console.log(vm.$data.fname); // must use function when in Vue.extend() var Component = Vue.extend({ data: function () { return _obj } }); var myComponentInstance = new Component(); console.log(myComponentInstance.lname); console.log(myComponentInstance.$data); </script> </body> </html>
對於組件,數據是一個函數,與Vue.extend一起使用,如上圖所示。數據是一個函數。例如,
data: function () { return _obj }
要引用組件中的數據,我們需要創建它的實例。例如,
var myComponentInstance = new Component();
要從數據中獲取詳細信息,我們需要像處理上面的父組件一樣進行操作。例如。
console.log(myComponentInstance.lname); console.log(myComponentInstance.$data);
以下是瀏覽器中顯示的詳細信息。
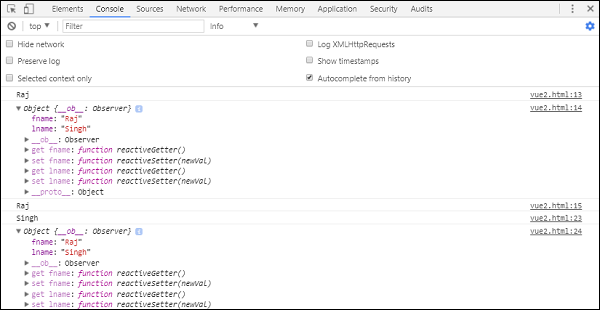
Props−Props的類型是字符串或對象的數組。它採用基於數組或基於對象的語法。它們被稱爲用於接受來自父組件的數據的屬性。
Example 1
Vue.component('props-demo-simple', { props: ['size', 'myMessage'] })
Example 2
Vue.component('props-demo-advanced', { props: { // just type check height: Number, // type check plus other validations age: { type: Number, default: 0, required: true, validator: function (value) { return value >= 0 } } } })
propsData−這用於單元測試。
鍵入字符串數組。例如,{[key:string]:any}。它需要在創建Vue實例期間傳遞。
Example
var Comp = Vue.extend({ props: ['msg'], template: '<div>{{ msg }}</div>' }) var vm = new Comp({ propsData: { msg: 'hello' } })
計算的−類型:{[key:string]:函數{get:Function,set:Function}
Example
<html> <head> <title>VueJs Introduction</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <script type = "text/javascript"> var vm = new Vue({ data: { a: 2 }, computed: { // get only, just need a function aSum: function () { return this.a + 2; }, // both get and set aSquare: { get: function () { return this.a*this.a; }, set: function (v) { this.a = v*2; } } } }) console.log(vm.aSquare); // -> 4 vm.aSquare = 3; console.log(vm.a); // -> 6 console.log(vm.aSum); // -> 8 </script> </body> </html>
Computed有兩個函數aSum和aSquare。
函數aSum只返回this.a+2。函數aSquare再次調用兩個函數:get和set。
變量vm是Vue的一個實例,它調用aSquare和aSum。另外vm.aSquare=3從aSquare調用set函數,vm.aSquare調用get函數。我們可以在瀏覽器中檢查輸出,如下截圖所示。
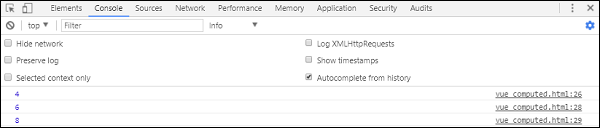
方法−方法將包含在Vue實例中,如以下代碼所示。我們可以使用Vue對象訪問函數。
<html> <head> <title>VueJs Introduction</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <script type = "text/javascript"> var vm = new Vue({ data: { a: 5 }, methods: { asquare: function () { this.a *= this.a; } } }) vm.asquare(); console.log(vm.a); // 25 </script> </body> </html>
方法是Vue構造函數的一部分。讓我們使用Vue對象調用方法vm.asquare(),屬性a的值在asquare函數中更新。a的值從1更改爲25,在下面的瀏覽器控制台中可以看到相同的值。