我們已經看到了Vue實例和組件的方法。計算性質類似於方法,但與我們將在本章討論的方法相比有一些差異。
在本章末尾,我們將能夠決定何時使用方法以及何時使用計算屬性。
讓我們用一個例子來理解計算屬性。
Example
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "computed_props"> FirstName : <input type = "text" v-model = "firstname" /> <br/><br/> LastName : <input type = "text" v-model = "lastname"/> <br/><br/> <h1>My name is {{firstname}} {{lastname}}</h1> <h1>Using computed method : {{getfullname}}</h1> </div> <script type = "text/javascript" src = "js/vue_computedprops.js"></script> </body> </html>
vue computeprops.js公司
var vm = new Vue({ el: '#computed_props', data: { firstname :"", lastname :"", birthyear : "" }, computed :{ getfullname : function(){ return this.firstname +" "+ this.lastname; } } })
在這裡,我們用firstname和lastname創建了.html文件。Firstname和Lastname是一個文本框,使用Firstname和Lastname屬性綁定。
我們調用計算方法getfullname,它返回輸入的名字和姓氏。
computed :{ getfullname : function(){ return this.firstname +" "+ this.lastname; } }
當我們在文本框中鍵入時,當屬性firstname或lastname更改時,函數將返回相同的結果。因此,在computed的幫助下,我們不必做任何特定的事情,比如記住調用函數。在computed中,當內部使用的屬性(即firstname和lastname)發生變化時,它會被自己調用。
以下瀏覽器中將顯示相同的內容。在文本框中鍵入,將使用computed函數對其進行更新。

現在,讓我們試著理解方法和計算屬性之間的區別。兩者都是物體。裡面有定義的函數,它返回一個值。
對於方法,我們將其作爲函數調用,並將其作爲屬性計算。使用下面的示例,讓我們了解方法和計算屬性之間的區別。
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "computed_props"> <h1 style = "background-color:gray;">Random No from computed property: {{getrandomno}}</h1> <h1>Random No from method: {{getrandomno1()}}</h1> <h1>Random No from method : {{getrandomno1()}}</h1> <h1 style = "background-color:gray;">Random No from computed property: {{getrandomno}}</h1> <h1 style = "background-color:gray;">Random No from computed property: {{getrandomno}}</h1> <h1 style = "background-color:gray;">Random No from computed property: {{getrandomno}}</h1> <h1>Random No from method: {{getrandomno1()}}</h1> <h1>Random No from method: {{getrandomno1()}}</h1> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#computed_props', data: { name : "helloworld" }, methods: { getrandomno1 : function() { return Math.random(); } }, computed :{ getrandomno : function(){ return Math.random(); } } }); </script> </body> </html>
在上面的代碼中,我們創建了一個名爲getrandomno1的方法和一個帶有函數getrandomno的計算屬性。兩者都使用Math.random()返回隨機數。
它顯示在瀏覽器中,如下所示。方法和計算屬性被多次調用以顯示差異。
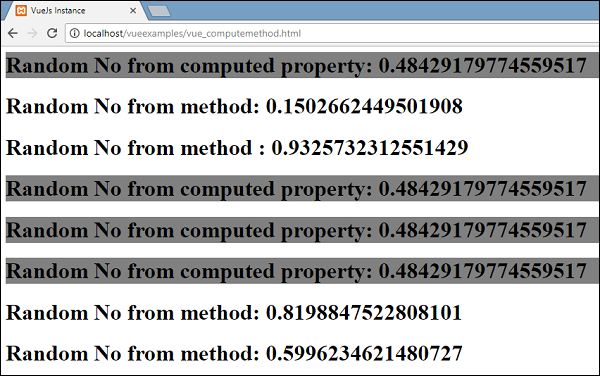
如果我們查看上面的值,我們將看到從computed屬性返回的隨機數保持不變,而不管它被調用的次數。這意味著每次調用它時,最後一個值都會更新。而對於一個方法,它是一個函數,因此,每次調用它都返回一個不同的值。
Get/Set in Computed Properties
在本節中,我們將通過一個示例了解計算屬性中的get/set函數。
Example
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "computed_props"> <input type = "text" v-model = "fullname" /> <h1>{{firstName}}</h1> <h1>{{lastName}}</h1> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#computed_props', data: { firstName : "Terry", lastName : "Ben" }, methods: { }, computed :{ fullname : { get : function() { return this.firstName+" "+this.lastName; } } } }); </script> </body> </html>
我們定義了一個輸入框,它綁定到全名,這是一個計算屬性。它返回一個名爲get的函數,該函數提供全名,即名字和姓氏。此外,我們還將firstname和lastname顯示爲−
<h1>{{firstName}}</h1> <h1>{{lastName}}</h1>
讓我們在瀏覽器中檢查一下。

現在,如果我們在文本框中更改名稱,我們將看到相同的內容不會反映在下面螢幕截圖中顯示的名稱中。
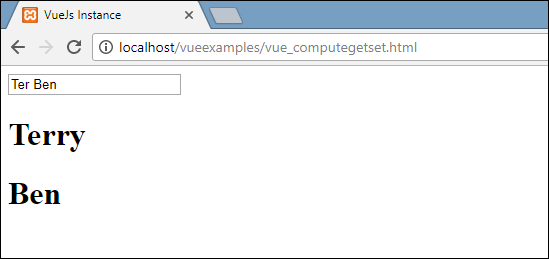
讓我們在fullname computed屬性中添加setter函數。
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "computed_props"> <input type = "text" v-model = "fullname" /> <h1>{{firstName}}</h1> <h1>{{lastName}}</h1> </div> <script type = "text/javascript"> var vm = new Vue({ el: '#computed_props', data: { firstName : "Terry", lastName : "Ben" }, methods: { }, computed :{ fullname : { get : function() { return this.firstName+" "+this.lastName; }, set : function(name) { var fname = name.split(" "); this.firstName = fname[0]; this.lastName = fname[1] } } } }); </script> </body> </html>
我們在fullname computed屬性中添加了set函數。
computed :{ fullname : { get : function() { return this.firstName+" "+this.lastName; }, set : function(name) { var fname = name.split(" "); this.firstName = fname[0]; this.lastName = fname[1] } } }
它將名稱作爲參數,這只是文本框中的全名。稍後,它將在空間上拆分,並更新firstname和lastname。現在,當我們運行代碼並編輯文本框時,瀏覽器中將顯示相同的內容。由於set函數,firstname和lastname將被更新。get函數返回firstname和lastname,而set函數則更新它(如果編輯了任何內容)。

現在,文本框中鍵入的內容與上面螢幕截圖中顯示的內容匹配。