C++字符串
C++提供了以下兩種類型表示字符串:
-
C風格的字符串
-
用標準C++引入了string類類型
C風格的字符串:
C語言風格的字符串源自C語言中,並繼續在C++中支持。字符串實際上是一個一維是由一個空字符'\0'終止字符數組。因此,一個空值終止字符串包含包括字符串後跟空字符。
下麵的聲明和初始化創建由單詞“Hello”的字符串。要在數組的末尾持有空字符,包含字符串的字符數組的大小小於字符在字的數量多了一個“Hello”。
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
如果按照數組初始化的規則,那麼可以寫上述聲明如下:
char greeting[] = "Hello";
以下是在C / C++以上定義字符串內存中表示:
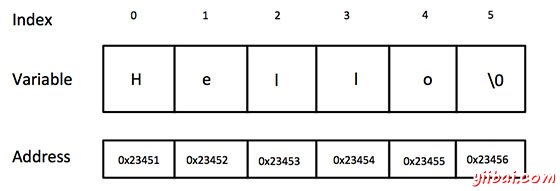
其實,不要把空字符的字符串常量的結尾。 C++編譯器自動將'\0'加在字符串的結尾,當它初始化數組。讓我們嘗試打印上麵提到的字符串:
#include <iostream> using namespace std; int main () { char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'}; cout << "Greeting message: "; cout << greeting << endl; return 0; }
當上述代碼被編譯和執行時,它會產生導致一些如下:
Greeting message: Hello
C++支持多種操縱空值終止字符串的函數:
S.N. | 功能及用途 |
---|---|
1 |
strcpy(s1, s2); 複製字符串s2到字符串s1 |
2 |
strcat(s1, s2); 連接字符串s2到字符串s1的末尾 |
3 |
strlen(s1); 返回字符串s1的長度 |
4 |
strcmp(s1, s2); 返回值為0,s1和s2是相同的; 如果s1<s2返回小於0;如果s1>s2 返回大於0 |
5 |
strchr(s1, ch); 返回一個指針,指向字符串s1中第一次出現字符ch |
6 |
strstr(s1, s2); 返回一個指針,指向字符串s1中第一次出現的字符串s2 |
下麵的例子使用小部分上述功能:
#include <iostream> #include <cstring> using namespace std; int main () { char str1[10] = "Hello"; char str2[10] = "World"; char str3[10]; int len ; // copy str1 into str3 strcpy( str3, str1); cout << "strcpy( str3, str1) : " << str3 << endl; // concatenates str1 and str2 strcat( str1, str2); cout << "strcat( str1, str2): " << str1 << endl; // total lenghth of str1 after concatenation len = strlen(str1); cout << "strlen(str1) : " << len << endl; return 0; }
當上述代碼被編譯和執行時,它會產生導致一些如下:
strcpy( str3, str1) : Hello strcat( str1, str2): HelloWorld strlen(str1) : 10
C++的String類:
標準C++庫提供了支持上述所有操作字符串類的類型,另外更多的功能。我們將C++標準庫中學習,但現在讓我們先來看看下麵的例子:
在這一點上,你可能不理解這個例子,因為到目前為止,我們還冇有討論類和對象。不過可以繼續,直到你有認識上麵的麵向對象的概念。
#include <iostream> #include <string> using namespace std; int main () { string str1 = "Hello"; string str2 = "World"; string str3; int len ; // copy str1 into str3 str3 = str1; cout << "str3 : " << str3 << endl; // concatenates str1 and str2 str3 = str1 + str2; cout << "str1 + str2 : " << str3 << endl; // total lenghth of str3 after concatenation len = str3.size(); cout << "str3.size() : " << len << endl; return 0; }
當上述代碼被編譯和執行時,它會產生導致一些如下:
str3 : Hello str1 + str2 : HelloWorld str3.size() : 10