web服務是一種基於web的功能,它使用web應用程式要使用的web協議來訪問。web服務開發有三個方面:
- Creating the web service
- Creating a proxy
- Consuming the web service
Creating a Web Service
web服務是一個web應用程式,它基本上是一個類,由其他應用程式可以使用的方法組成。它還遵循代碼隱藏架構(如ASP.NET網頁),儘管它沒有用戶界面。
爲了理解這個概念,讓我們創建一個web服務來提供股票價格信息。客戶可以根據股票符號查詢股票的名稱和價格。爲了使這個例子簡單,這些值在二維數組中硬編碼。此web服務有三種方法:
- A default HelloWorld method
- A GetName Method
- A GetPrice Method
請執行以下步驟創建web服務:
步驟(1):在Visual Studio中選擇「文件」->「新建」->「網站」,然後選擇「ASP.NET Web服務」。
步驟(2):在項目的App_code目錄中創建名爲service.asmx的web服務文件及其代碼隱藏文件service.cs。
步驟(3):將文件名更改爲StockService.asmx和StockService.cs。
步驟(4):asmx文件上只有一個WebService指令:
<%@ WebService Language="C#" CodeBehind="~/App_Code/StockService.cs" Class="StockService" %>
步驟(5):打開StockService.cs文件,其中生成的代碼是基本的Hello World服務。默認的web服務代碼隱藏文件如下所示:
using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Linq; using System.Web; using System.Web.Services; using System.Web.Services.Protocols; using System.Xml.Linq; namespace StockService { // <summary> // Summary description for Service1 // <summary> [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] [ToolboxItem(false)] // To allow this Web Service to be called from script, // using ASP.NET AJAX, uncomment the following line. // [System.Web.Script.Services.ScriptService] public class Service1 : System.Web.Services.WebService { [WebMethod] public string HelloWorld() { return "Hello World"; } } }
步驟(6):更改代碼隱藏文件以添加股票符號、名稱和價格的二維字符串數組以及獲取股票信息的兩個web方法。
using System; using System.Linq; using System.Web; using System.Web.Services; using System.Web.Services.Protocols; using System.Xml.Linq; [WebService(Namespace = "http://tempuri.org/")] [WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)] // To allow this Web Service to be called from script, // using ASP.NET AJAX, uncomment the following line. // [System.Web.Script.Services.ScriptService] public class StockService : System.Web.Services.WebService { public StockService () { //Uncomment the following if using designed components //InitializeComponent(); } string[,] stocks = { {"RELIND", "Reliance Industries", "1060.15"}, {"ICICI", "ICICI Bank", "911.55"}, {"JSW", "JSW Steel", "1201.25"}, {"WIPRO", "Wipro Limited", "1194.65"}, {"SATYAM", "Satyam Computers", "91.10"} }; [WebMethod] public string HelloWorld() { return "Hello World"; } [WebMethod] public double GetPrice(string symbol) { //it takes the symbol as parameter and returns price for (int i = 0; i < stocks.GetLength(0); i++) { if (String.Compare(symbol, stocks[i, 0], true) == 0) return Convert.ToDouble(stocks[i, 2]); } return 0; } [WebMethod] public string GetName(string symbol) { // It takes the symbol as parameter and // returns name of the stock for (int i = 0; i < stocks.GetLength(0); i++) { if (String.Compare(symbol, stocks[i, 0], true) == 0) return stocks[i, 1]; } return "Stock Not Found"; } }
步驟(7):運行web服務應用程式將提供一個web服務測試頁,該頁允許測試服務方法。
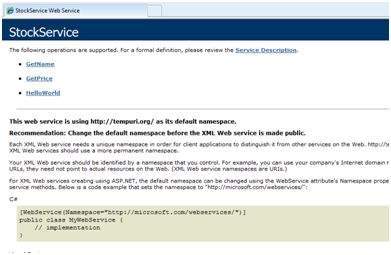
步驟(8):單擊方法名,檢查它是否正常運行。
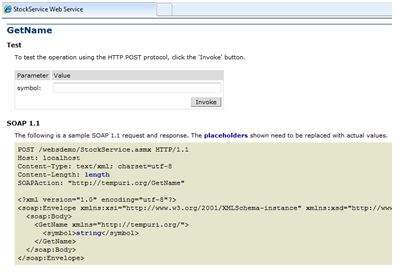
步驟(9):要測試GetName方法,請提供一個經過硬編碼的股票符號,它將返回股票的名稱
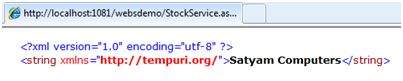
Consuming the Web Service
要使用web服務,請在同一解決方案下創建網站。這可以通過右鍵單擊解決方案資源管理器中的解決方案名稱來完成。調用web服務的web頁面應該有一個label控制項來顯示返回的結果,還有兩個按鈕控制項,一個用於回發,另一個用於調用服務。
web應用程式的內容文件如下:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="wsclient._Default" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" > <head runat="server"> <title> Untitled Page </title> </head> <body> <form id="form1" runat="server"> <div> <h3>Using the Stock Service</h3> <br /> <br /> <asp:Label ID="lblmessage" runat="server"></asp:Label> <br /> <br /> <asp:Button ID="btnpostback" runat="server" onclick="Button1_Click" Text="Post Back" style="width:132px" /> <asp:Button ID="btnservice" runat="server" onclick="btnservice_Click" Text="Get Stock" style="width:99px" /> </div> </form> </body> </html>
web應用程式的代碼隱藏文件如下:
using System; using System.Collections; using System.Configuration; using System.Data; using System.Linq; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.HtmlControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Xml.Linq; //this is the proxy using localhost; namespace wsclient { public partial class _Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { lblmessage.Text = "First Loading Time: " + DateTime.Now.ToLongTimeString } else { lblmessage.Text = "PostBack at: " + DateTime.Now.ToLongTimeString(); } } protected void btnservice_Click(object sender, EventArgs e) { StockService proxy = new StockService(); lblmessage.Text = String.Format("Current SATYAM Price:{0}", proxy.GetPrice("SATYAM").ToString()); } } }
Creating the Proxy
代理是web服務代碼的代理。在使用web服務之前,必須創建代理。代理已在客戶端應用程式中註冊。然後客戶端應用程式使用本地方法調用web服務。
代理接受調用,將其包裝成適當的格式,並將其作爲SOAP請求發送到伺服器。SOAP代表簡單對象訪問協議。此協議用於交換web服務數據。
當伺服器將SOAP包返回給客戶端時,代理將解碼所有內容並將其呈現給客戶端應用程式。
在使用btnservice_Click調用web服務之前,應該向應用程式添加一個web引用。這將透明地創建一個代理類,由btnservice_Click事件使用。
protected void btnservice_Click(object sender, EventArgs e) { StockService proxy = new StockService(); lblmessage.Text = String.Format("Current SATYAM Price: {0}", proxy.GetPrice("SATYAM").ToString()); }
按照以下步驟創建代理:
步驟(1):右鍵單擊解決方案資源管理器中的web應用程式條目,然後單擊「添加web引用」。
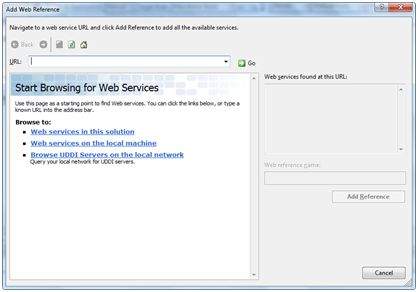
步驟(2):選擇「此解決方案中的Web服務」。它返回StockService引用。
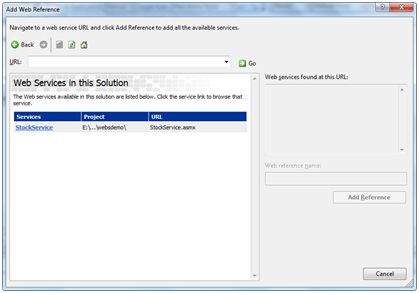
步驟(3):單擊服務將打開測試網頁。默認情況下,創建的代理稱爲「localhost」,您可以重命名它。單擊「添加引用」將代理添加到客戶端應用程式。
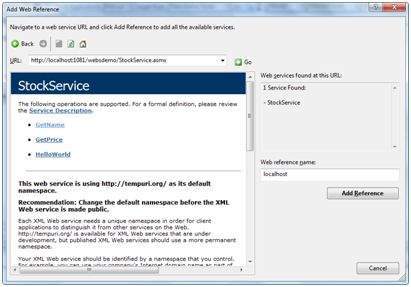
通過添加以下內容將代理包含在代碼隱藏文件中: