JSP語法
本教學將給予基本概念上簡單的語法(即元素)涉及JSP開發:
Scriptlet腳本:
其中腳本可以包含任意數量的JAVA語言的語句,變量或方法聲明或表達式是有效的網頁腳本語言。
以下是腳本的語法:
<% code fragment %>
可以編寫XML相當於上麵的語法如下:
<jsp:scriptlet> code fragment </jsp:scriptlet>
任何文本,HTML標記,或者JSP元素你寫必須在scriptlet的外麵。下麵是一個簡單的和第一個例子的JSP:
<html> <head><title>Hello World</title></head> <body> Hello World!<br/> <% out.println("Your IP address is " + request.getRemoteAddr()); %> </body> </html>
注意:假設Apache Tomcat的安裝在C:apache-tomcat-7.0.2 和 環境設置為每個教學設置環境。
讓我們繼續上麵的代碼在JSP文件hello.jsp中,並把這個文件在C:apache-tomcat-7.0.2webappsROOT目錄,並嘗試通過給網址http://localhost:8080/hello.jsp瀏覽它。這將產生以下結果:
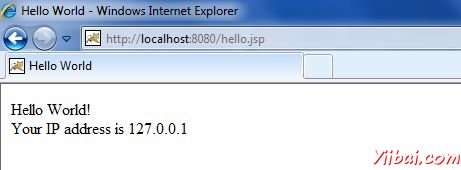
JSP 聲明:
聲明一個或多個變量,或者你可以在Java代碼後在JSP文件中使用的方法。在JSP文件中使用它之前,必須聲明的變量或方法。
以下是JSP聲明的語法:
<%! declaration; [ declaration; ]+ ... %>
您可以編寫XML相當於上麵的語法如下:
<jsp:declaration> code fragment </jsp:declaration>
下麵是一個簡單的例子JSP聲明:
<%! int i = 0; %> <%! int a, b, c; %> <%! Circle a = new Circle(2.0); %>
JSP 表達式:
JSP表達式元素包含被評估的腳本語言表達,轉化為字符串,並在表達出現在JSP文件中插入。
因為一個表達式的值轉換為String,您可以在一行文本中使用表達式,它是否被標記為HTML,在JSP文件中。
表達式的元素可以包含根據Java語言規範是任何有效的表達式,但你不能用一個分號來結束的表達式。
以下是JSP表達式的語法:
<%= expression %>
您可以編寫XML相當於上麵的語法如下:
<jsp:expression> expression </jsp:expression>
下麵是一個簡單的例子JSP表達式:
<html> <head><title>A Comment Test</title></head> <body> <p> Today's date: <%= (new java.util.Date()).toLocaleString()%> </p> </body> </html>
這將產生以下結果:
Today's date: 11-Sep-2010 21:24:25 |
JSP的注釋:
JSP注釋標記的文字或說明JSP容器應忽略。當你想要隱藏或“注釋掉”你的JSP頁麵的一部分,一個JSP注釋是很有用的。
以下是JSP注釋的語法:
<%-- This is JSP comment --%>
下麵是一個簡單的例子JSP評論:
<html> <head><title>A Comment Test</title></head> <body> <h2>A Test of Comments</h2> <%-- This comment will not be visible in the page source --%> </body> </html>
這將產生以下結果:
A Test of Comments |
有少數特殊的結構可以用在各種情況下插入注釋,否則將被特殊處理的字符。這裡有一個總結:
語法 | 目的 |
---|---|
<%-- comment --%> | A JSP comment. Ignored by the JSP engine. |
<!-- comment --> | An HTML comment. Ignored by the browser. |
<\% | Represents static <% literal. |
%> | Represents static %> literal. |
' | A single quote in an attribute that uses single quotes. |
" | A double quote in an attribute that uses double quotes. |
JSP指令:
一個JSP指令影響Servlet類的整體結構。它通常具有以下形式:
<%@ directive attribute="value" %>
有三種類型的指令標簽:
指令 | 描述 |
---|---|
<%@ page ... %> | Defines page-dependent attributes, such as scripting language, error page, and buffering requirements. |
<%@ include ... %> | Includes a file during the translation phase. |
<%@ taglib ... %> | Declares a tag library, containing custom actions, used in the page |
我們將解釋JSP指令在單獨的一章 JSP - 指令
JSP 動作:
JSP動作使用XML語法結構來控製Servlet引擎的行為。您可以動態地插入文件,重用JavaBeans組件,用戶轉發到另一個頁麵,或生成HTML的Java插件。
隻有一個用於操作元素語法,因為它符合XML標準:
<jsp:action_name attribute="value" />
動作要素基本上都是預定義的功能,而且已有下麵的JSP操作:
語法 | 目的 |
---|---|
jsp:include | Includes a file at the time the page is requested |
jsp:include | Includes a file at the time the page is requested |
jsp:useBean | Finds or instantiates a JavaBean |
jsp:setProperty | Sets the property of a JavaBean |
jsp:getProperty | Inserts the property of a JavaBean into the output |
jsp:forward | Forwards the requester to a new page |
jsp:plugin | Generates browser-specific code that makes an OBJECT or EMBED tag for the Java plugin |
jsp:element | Defines XML elements dynamically. |
jsp:attribute | Defines dynamically defined XML element's attribute. |
jsp:body | Defines dynamically defined XML element's body. |
jsp:text | Use to write template text in JSP pages and documents. |
我們將解釋在單獨的章節JSP動作 JSP 動作
JSP隱含對象:
JSP支持九種自動定義的變量,這也被稱為隱式對象。這些變量是:
對象 | 描述 |
---|---|
request | This is the HttpServletRequest object associated with the request. |
response | This is the HttpServletResponse object associated with the response to the client. |
out | This is the PrintWriter object used to send output to the client. |
session | This is the HttpSession object associated with the request. |
application | This is the ServletContext object associated with application context. |
config | This is the ServletConfig object associated with the page. |
pageContext | This encapsulates use of server-specific features like higher performance JspWriters. |
page | This is simply a synonym for this, and is used to call the methods defined by the translated servlet class. |
Exception | The Exception object allows the exception data to be accessed by designated JSP. |
我們將解釋在單獨的一章JSP隱含對象JSP隱含對象.
控製流語句:
JSP提供了Java的所有功能被嵌入在Web應用程序。可以使用所有的API和Java的構建模塊在JSP程序,包括決策語句,循環等。
決策語句:
if...else 塊開始時像一個普通的Scriptlet,但Scriptlet是在包括scriptlet標記之間的HTML文本的每一行封閉。
<%! int day = 3; %> <html> <head><title>IF...ELSE Example</title></head> <body> <% if (day == 1 | day == 7) { %> <p> Today is weekend</p> <% } else { %> <p> Today is not weekend</p> <% } %> </body> </html>
這將產生以下結果:
Today is not weekend |
查看以下語句 switch...case已經寫了一個有點不同使用塊out.println() 和內嵌 Scriptletas:
<%! int day = 3; %> <html> <head><title>SWITCH...CASE Example</title></head> <body> <% switch(day) { case 0: out.println("It's Sunday."); break; case 1: out.println("It's Monday."); break; case 2: out.println("It's Tuesday."); break; case 3: out.println("It's Wednesday."); break; case 4: out.println("It's Thursday."); break; case 5: out.println("It's Friday."); break; default: out.println("It's Saturday."); } %> </body> </html>
這將產生以下結果:
It's Wednesday. |
循環語句:
您還可以使用三種基本類型的Java循環塊:for, while,and do…while 塊在JSP編程。
讓我們看看下麵的for循環的例子:
<%! int fontSize; %> <html> <head><title>FOR LOOP Example</title></head> <body> <%for ( fontSize = 1; fontSize <= 3; fontSize++){ %> <font color="green" size="<%= fontSize %>"> JSP Tutorial </font><br /> <%}%> </body> </html>
這將產生以下結果:
JSP Tutorial JSP Tutorial JSP Tutorial |
上麵的例子中可以使用while循環如下這樣寫:
<%! int fontSize; %> <html> <head><title>WHILE LOOP Example</title></head> <body> <%while ( fontSize <= 3){ %> <font color="green" size="<%= fontSize %>"> JSP Tutorial </font><br /> <%fontSize++;%> <%}%> </body> </html>
這也將產生以下結果:
JSP Tutorial JSP Tutorial JSP Tutorial |
JSP 運算符:
JSP支持所有Java支持的邏輯和算術運算符。下表給出所有的運算符具有最高優先級的列表顯示在表的頂部,最低的在底部。
在表達式中,優先級較高的運算符將首先計算。
分類 | 運算符 | 關聯 |
---|---|---|
Postfix | () [] . (dot operator) | Left to right |
Unary | ++ - - ! ~ | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | >> >>> << | Left to right |
Relational | > >= < <= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %= >>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
JSP常值:
JSP表達式語言定義了以下常量:
-
Boolean: true and false
-
Integer: as in Java
-
Floating point: as in Java
-
String: with single and double quotes; " is escaped as ", ' is escaped as ', and is escaped as \.
-
Null: null