Swing JProgressBar
JProgressBar類是一個組件,它可視地顯示某些任務的進度。
類聲明
以下是聲明 javax.swing.JProgressBar類:
public class JProgressBar extends JComponent implements SwingConstants, Accessible
字段域
以下是javax.swing.JProgressBar類的字段域:
-
protected ChangeEvent changeEvent --因為事件的唯一有趣的屬性是不可改變的源,這是進度條,需要每個實例隻有一個ChangeEvent事件。
-
protected ChangeListener changeListener --監聽發送進度條的模型的變化事件,他們重新調度變更登記的的事件監聽器後。
-
protected BoundedRangeModel model --對象保存數據進度條。
-
protected int orientation --無論是進度條是水平或垂直。
-
protected boolean paintBorder --是否顯示進度條周圍的邊框。
-
protected boolean paintString --無論在進度條上顯示的文本字符串。
-
protected String progressString --可以在進度條上顯示的可選字符串。
類構造函數
S.N. | 構造函數 & 描述 |
---|---|
1 |
JProgressBar() Creates a horizontal progress bar that displays a border but no progress string. |
2 |
JProgressBar(BoundedRangeModel newModel) Creates a horizontal progress bar that uses the specified model to hold the progress bar's data. |
3 |
JProgressBar(int orient) Creates a progress bar with the specified orientation, which can be either SwingConstants.VERTICAL or SwingConstants.HORIZONTAL. |
4 |
JProgressBar(int min, int max) Creates a horizontal progress bar with the specified minimum and maximum. |
5 |
JProgressBar(int orient, int min, int max) Creates a progress bar using the specified orientation, minimum, and maximum. |
類方法
S.N. | 方法 & 描述 |
---|---|
1 |
void addChangeListener(ChangeListener l) Adds the specified ChangeListener to the progress bar. |
2 |
protected ChangeListener createChangeListener() Subclasses that want to handle change events from the model differently can override this to return an instance of a custom ChangeListener implementation. |
3 |
protected void fireStateChanged() Send a ChangeEvent, whose source is this JProgressBar, to all ChangeListeners that have registered interest in ChangeEvents. |
4 |
AccessibleContext getAccessibleContext() Gets the AccessibleContext associated with this JProgressBar. |
5 |
ChangeListener[] getChangeListeners() Returns an array of all the ChangeListeners added to this progress bar with addChangeListener. |
6 |
int getMaximum() Returns the progress bar's maximum value from the BoundedRangeModel. |
7 |
int getMinimum() Returns the progress bar's minimum value from the BoundedRangeModel. |
8 |
BoundedRangeModel getModel() Returns the data model used by this progress bar. |
9 |
int getOrientation() Returns SwingConstants.VERTICAL or SwingConstants.HORIZONTAL, depending on the orientation of the progress bar. |
10 |
double getPercentComplete() Returns the percent complete for the progress bar. |
11 |
String getString() Returns a String representation of the current progress. |
12 |
ProgressBarUI getUI() Returns the look-and-feel object that renders this component. |
13 |
String getUIClassID() Returns the name of the look-and-feel class that renders this component. |
14 |
int getValue() Returns the progress bar's current value from the BoundedRangeModel. |
15 |
boolean isBorderPainted() Returns the borderPainted property. |
16 |
boolean isIndeterminate() Returns the value of the indeterminate property. |
17 |
boolean isStringPainted() Returns the value of the stringPainted property. |
18 |
protected void paintBorder(Graphics g) Paints the progress bar's border if the borderPainted property is true. |
19 |
protected String paramString() Returns a string representation of this JProgressBar. |
20 |
void removeChangeListener(ChangeListener l) Removes a ChangeListener from the progress bar. |
21 |
void setBorderPainted(boolean b) Sets the borderPainted property, which is true if the progress bar should paint its border. |
22 |
void setIndeterminate(boolean newValue) Sets the indeterminate property of the progress bar, which determines whether the progress bar is in determinate or indeterminate mode. |
23 |
void setMaximum(int n) Sets the progress bar's maximum value (stored in the progress bar's data model) to n. |
24 |
void setMinimum(int n) Sets the progress bar's minimum value (stored in the progress bar's data model) to n. |
25 |
void setModel(BoundedRangeModel newModel) Sets the data model used by the JProgressBar. |
26 |
void setOrientation(int newOrientation) Sets the progress bar's orientation to newOrientation, which must be SwingConstants.VERTICAL or SwingConstants.HORIZONTAL. |
27 |
void setString(String s) Sets the value of the progress string. |
28 |
void setStringPainted(boolean b) Sets the value of the stringPainted property, which determines whether the progress bar should render a progress string. |
29 |
void setUI(ProgressBarUI ui) Sets the look-and-feel object that renders this component. |
30 |
void setValue(int n) Sets the progress bar's current value to n. |
31 |
void updateUI() Resets the UI property to a value from the current look and feel. |
方法繼承
這個類從以下類繼承的方法:
-
javax.swing.JComponent
-
java.awt.Container
-
java.awt.Component
-
java.lang.Object
JProgressBar 例子
選擇使用任何編輯器創建以下java程序在 D:/ > SWING > com > yiibai > gui >
SwingControlDemo.javapackage com.yiibai.gui; import java.awt.*; import java.awt.event.*; import javax.swing.*; public class SwingControlDemo { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; public SwingControlDemo(){ prepareGUI(); } public static void main(String[] args){ SwingControlDemo swingControlDemo = new SwingControlDemo(); swingControlDemo.showProgressBarDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Java Swing Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new JLabel("", JLabel.CENTER); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,100); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private JProgressBar progressBar; private Task task; private JButton startButton; private JTextArea outputTextArea; private void showProgressBarDemo(){ headerLabel.setText("Control in action: JProgressBar"); progressBar = new JProgressBar(0, 100); progressBar.setValue(0); progressBar.setStringPainted(true); startButton = new JButton("Start"); outputTextArea = new JTextArea("",5,20); JScrollPane scrollPane = new JScrollPane(outputTextArea); startButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { task = new Task(); task.start(); }}); controlPanel.add(startButton); controlPanel.add(progressBar); controlPanel.add(scrollPane); mainFrame.setVisible(true); } private class Task extends Thread { public Task(){ } public void run(){ for(int i =0; i<= 100; i+=10){ final int progress = i; SwingUtilities.invokeLater(new Runnable() { public void run() { progressBar.setValue(progress); outputTextArea.setText(outputTextArea.getText() + String.format("Completed %d%% of task. ", progress)); } }); try { Thread.sleep(100); } catch (InterruptedException e) {} } } } }
編譯程序,使用命令提示符。到 D:/ > SWING 然後輸出以下命令。
D:SWING>javac comyiibaiguiSwingControlDemo.java
如果冇有錯誤出現,這意味著編譯成功。使用下麵的命令來運行程序。
D:SWING>java com.yiibai.gui.SwingControlDemo
驗證下麵的輸出
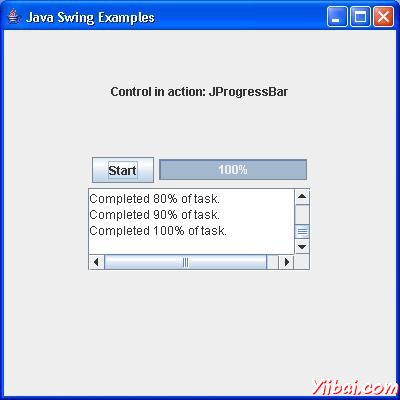